Hello Friends, Today we are going to build a simple words dictionary using python and a json file which contains words. Dictionary can help you to get meanings and details about some words and also it can provide you suggestions if similar words search.
First of all we need to import as below
import json from difflib import get_close_matches # this is to get closer match from list
Reference : https://docs.python.org/3/library/difflib.html
Once our import is done you will require a json file which contains all words. you can download it from here
# load data from json file data = json.load(open('data.json'))
We can use json library provided by python to load a json file as above mentioned code. it will create data object which has all json file data loaded and parsed. now let’s work on actual dictionary part.
# function which will provide information def translate(w): if w in data: return data[w] elif len(get_close_matches(w, data.keys())) > 0: print("Did you mean %s instead? " % get_close_matches(w, data.keys())[0]) ans = input('Enter y or n:') if ans.lower() == 'y': return data[get_close_matches(w, data.keys())[0]] else: return "did not find that word" else: return "The word doesn't exist. please double check it."
We have created function with name translate which takes w (word) as input and check if our word is present in our data object (as a key) if that’s true it will return details from dictionary.
or it will use get_close_matches function to fetch similar words from dictionary and ask user input if we mean any of those words, if we press yes it will provide the best match and return it as output else it will show that no word found. Now lets’ take input from user which word
# input from user word = input('Enter Word you want to search : ')
So we are almost done with our logic , input and output part. now let’s run code using below function.
# call function output = translate(word.lower()) if type(output) == list: # we are checking if it returns list or word for item in output: print("-> " + item) else: print(output)
Finally whole code will look like this as shown below
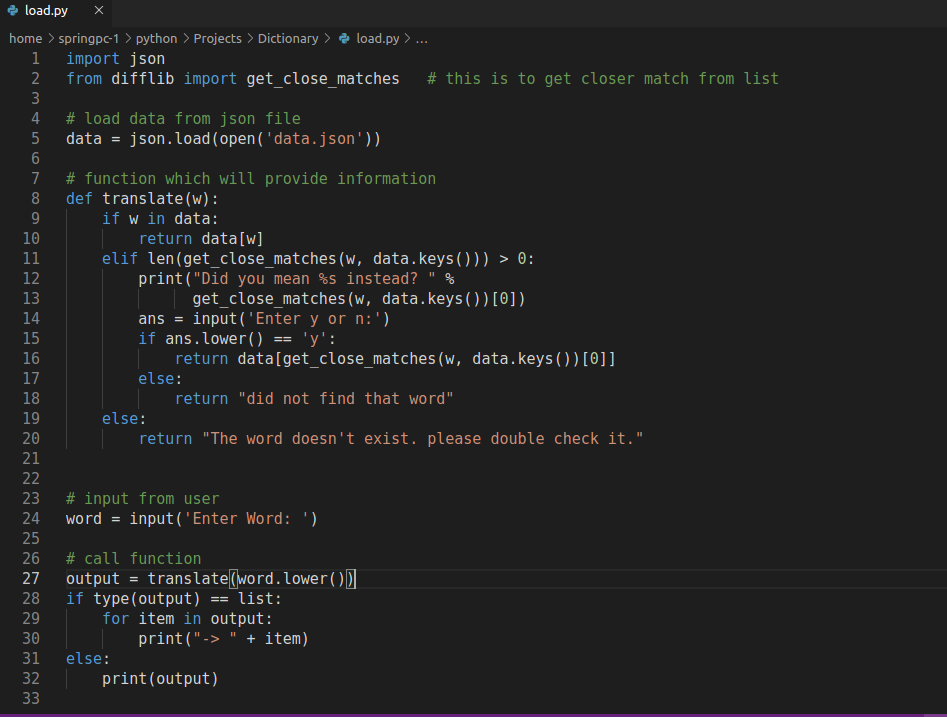
Great, Now let’s check output of above code
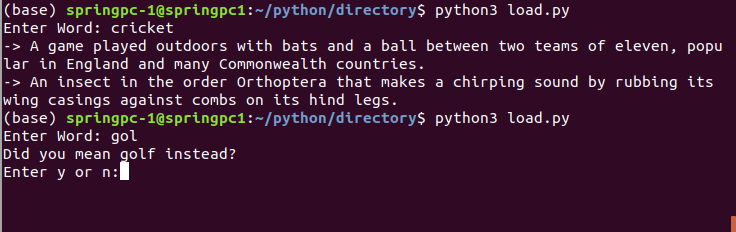