Face detection is one of the fundamental applications used in face recognition technology. Big companies like Facebook , amazon and Google has developed their software for face recognition but before they can recognize a face, their software must be able to detect face first from image.
In this project, We have applied face detection to some photos using OpenCV with Python. OpenCV is open source software library that allow developers to access routines in API used for computer vision applications.
First you need to install OpenCV using pip
pip install opencv-python
Once OpenCV is installed we required haar cascades file, Haar cascade uses machine learning techniques in which a function is trained from lot of positive and negative images. This process in algorithm is feature extraction. Training data used in this project is an XML file called haarcascade_frontalface_default.xml
Read more about Haar Cascade :
https://docs.opencv.org/3.3.0/d7/d8b/tutorial_py_face_detection.html
https://github.com/opencv/opencv/tree/master/data/haarcascades
For this project we have prepared directory where we have downloaded required files which include haar cascade file . images and face_detection.py file
# Load opencv library import cv2 # load face_cascade from haarcascade face xml file face_cascade = cv2.CascadeClassifier("haarcascade_frontalface_default.xml") # take image as input img = cv2.imread("multiface.jpg") # convert image into gray scale gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # detect faces faces = face_cascade.detectMultiScale( gray_img, scaleFactor=1.1, minNeighbors=10) # create list of faces and make rectangle around faces for x, y, w, h in faces: img = cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 3) # resize image to fit resized_img = cv2.resize(img, (int(img.shape[1]/2), int(img.shape[0]/2))) # show image cv2.imshow("gray", resized_img) cv2.waitKey(0) cv2.destroyAllWindows()
Output :
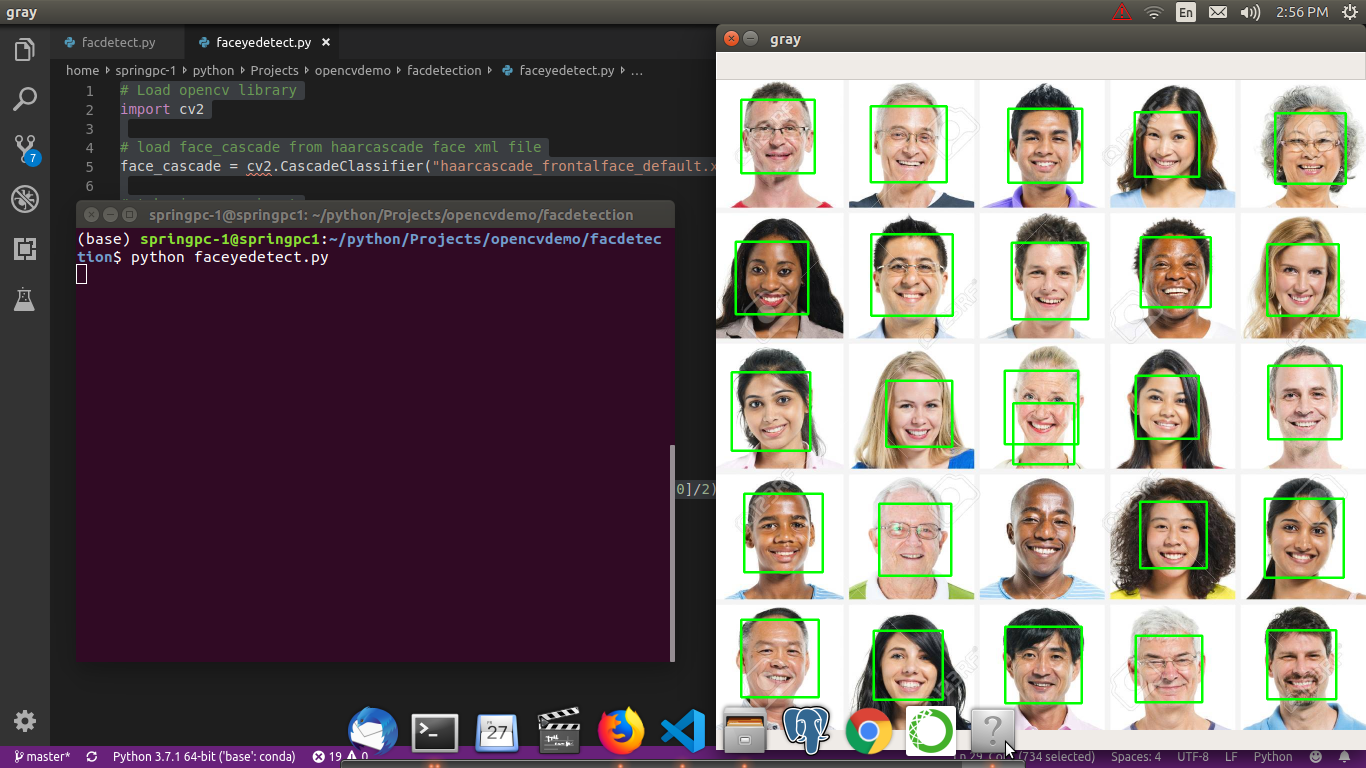
Looks like we got 90% results out of image but still this results lead to no conclusion. The code does not automatically tune parameters and provide best results. We tried with some non human faces and this code can not detect it. This is therefore not a fully intelligent system since it requires iteration from the user.
Refer code : https://github.com/akashsenta13/opencvdemo/tree/master/facdetection